package com.javaWave.blogSpot;
public class String2double {
/**
* @param args
*/
public static void main(String[] args) {
// String myString = "javaWave"; // do this if you want an exception
String myString = "100.00";
try {
double convertedValue =
Double.valueOf(myString.trim()).doubleValue();
System.out.println("convertedValue = " + convertedValue);
} catch (NumberFormatException nfe) {
System.out.println("NumberFormatException: "
+ nfe.getMessage());
}
}
}
javawaveblogs-20
Tuesday, December 2, 2008
Converting Java String to double
Sunday, August 10, 2008
Saturday, July 26, 2008
Core Java Notes- Part I
Think about objects created from the class.
· Things the object knows about itself-> instance variable
· Things the object does-> methods.
Note: think of instance as another way of saying object.
Difference between a class and a object:
Note: a class is not an object
->class is used to construct an object
A class is an blueprint for an object
-> tells the virtual machine how to make an object of the particular type.
Example: An object is like one entry in your address book.
The two uses of main:
1. To test your real class.
2. To launch/ start your java application.
The Heap
Each time an object is created in java, it goes into an area of memory known as the Heap. All objects created live on the heap.
Note: The java heap is actually called as Garbage collectable heap.
Java manages the memory for you. When the JVM can see that an object can never be used again, that object becomes eligible for garbage collection. And if you are running low on memory, the garbage collector will run, throw out the unreachable objects, and free up the space, so that the space can be reused.
Marking a method as public and static:
Marking a method as public and static makes it behave much like a ‘global’.
Note: Any code in any class of your application can access a public static method.
If you mark a variable as public, static and final, you have essentially made a globally available constant.
Variables
2 flavours of variables,
· Primitive
· Reference
Primitive: Hold fundamental values including integers, Booleans and floating point numbers.
Object references: hold, well, references to objects.
Two declare a variable you must follow two rules:
1. variables must have a type.
2. variables must have a name.
Example:
int count
here,
int ==> Type, and
count ==> Name.
Note: A variable is just a container that holds something.
You can assign a value to a variable in one of several ways including:
> Type a literal value after the equal sign, eg., x=12, isgod=true; etc.,
> assign the value of one variable to another (x=y).
> use an expression combining the two. Eg.(x=y+43).
Note: You need a name and a type for your variables
int size = 32
here ,
int ==> Type,
size ==> Name, and
32 ==> Literal
Safe naming rules for a class method or variable:
· it must start with a letter, underscore(_), or dollar sign($).you can’t start a name with a number.
· After the first character you can use the number as well.
· It can be anything you like, subject to those two rules, just so long as it isn’t one of java’s reserved words.
Reserved words Table:

There is actually no such thing as an object variables
There is only an object reference variable.
An object reference variable holds bits that representation way to access an object. And the JVM knows how to use the reference to get to the object.
Note: Objects live in one place-the garbage collectible heap!
Arrays: Arrays are always objects, whether they are declared to hold primitives or object references.
Note: once you have declared an array, you can’t put anything in it except things that are of the declared array type.
Bullet Points:
Variables come in two flavours,
1. Primitive
2. Reference
Variables must always be declared with a name and a type.
A primitive variable value is the bits representing a way to get to an object on the heap.
A reference variable is like a remote control using the dot operator (.) on a reference variable is like pressing a button on the remote control to access a method or instance variable.
A reference variable has a value of null when it is not referencing any object.
An array is always an object, even if the array is declared to hold primitives. There is no such thing as a primitive array, only an array that holds primitives
Note: Java is pass-by-value (i.e) pass-by-copy.
Bullet Points:
· Classes define what an object knows and what an object does.
· Things an object knows are its instance variables(state).
· Things an object does are its methods(behaviour).
· Methods can use instance variables so that objects of the same type can behave differently.
· A method can have parameters, which means you can pass one or more values into the method.
· The number and type of values you pass in must match the order and type of the parameters declared by the method.
· Values passed in and out of methods can be implicitly promoted to a larger type or explicitly cast to a smaller type.
· The value you pass as an argument to a method can be a literal value (2, ‘c’,etc) or a variable of the declared parameter type (for example, x where x is an int variable).
· A method must declare a return type. A void return type means the method doesn’t return type.
Encapsulation ==> Hide the data:
Rule of thumb: Mark your instance variables private and provide public getters and setters for access control.
The difference between instance and local variable.
instance variable are declared inside a class but not within a method.
local variables are declared within a method.
local variables must be initialized before use.
Note: Local variables do not get a default value! The compiler complains if you try to use a local variable before the variable is initialized.
Note: Method parameters are virtually the same as local variables. But method parameters will never get a compiler error telling you that a parameter variable might not have been initialized.
Monday, April 14, 2008
Quartz Job Scheduler -- Part II (Example, Simple Trigger)
Our application will just print Hello World on console after specified time.
For implementing the scheduler using quartz we need two classes.
1. which will implement org.quartz.Job interface, and the other
2. the scheduler class which will start the scheduler.
Now we will see the code which will implement Job interface:
package com.MyQuartz.simple;
import java.util.Date;
import org.quartz.Job;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
/**
*
* @author dhanago
*/
public class HelloJob implements Job {
public void execute(JobExecutionContext jobExecutionContext)
throws JobExecutionException {
System.out.println("Hello World -- Executed on : " + new Date());
}
}
Here,
execute() --> is an overridden method. When ever Job interface is implemented its execute() of method should be overridden. Note that any component you want to schedule should implement Job interface.
JobExecutionContext --> is passed as an parameter to the execute() method. this provides the job instance which provides the job instance with information about its run-time environment. From this we will get the job detail information and also some important information regarding its triggers etc.,
Now we will see the code which will start the scheduler:
package com.MyQuartz.simple;
import java.util.Date;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.quartz.JobDetail;
import org.quartz.Scheduler;
import org.quartz.SchedulerException;
import org.quartz.SimpleTrigger;
import org.quartz.impl.StdSchedulerFactory;
/**
*
* @author dhanago
*/
public class StartScheduler {
public void startScheduler()
throws SchedulerException {
Scheduler scheduler = new StdSchedulerFactory().getScheduler();
scheduler.start();
JobDetail jobDetail = new JobDetail(
"MyJob", scheduler.DEFAULT_GROUP, HelloJob.class);
SimpleTrigger simpleTrigger = new SimpleTrigger(
"MyTrigger", scheduler.DEFAULT_GROUP, new Date(),
null, SimpleTrigger.REPEAT_INDEFINITELY, 60L * 1000L);
scheduler.scheduleJob(jobDetail, simpleTrigger);
}
public static void main(String args[]) {
StartScheduler startScheduler = new StartScheduler();
try {
startScheduler.startScheduler();
}
catch (SchedulerException ex) {
Logger.getLogger(StartScheduler.class.getName()).
log(Level.SEVERE, null, ex);
}
}
}
Here,
StdSchedulerFactory(): A Class StdSchedulerFactory is a class and it is implementation of SchedulerFactory interface. Here it just using for create an instance of SchedulerFactory instance.
Scheduler: Scheduler interface is the main interface (API) to this functionality. It provides some simple operations like scheduling jobs, unscheduling jobs, starting/stopping/pausing the scheduler.
start(): This method is used to starts the Scheduler's threads that fire Triggers. At the first time when we create the Scheduler it is in "stand-by" mode, and will not fire triggers. The scheduler can also be send back into stand-by mode by invoking the standby() method.
JobDetail(String name, String group, Class jobclass): The JobDetail object is created at the time the Job is added to scheduler. It contains various property settings like job name, group name and job class name. It can be used to store state information for a given instance of job class.
SimpleTrigger(String name, String group, Date startTime, Date endTime, int repeatCount, long repeatInterval): Trigger objects are used to firing the execution of jobs. When you want to schedule the job, instantiate the trigger and set the properties to provide the scheduling.
DEFAULT_GROUP: It is a constant, specified that Job and Trigger instances are belongs to which group..
REPEAT_INDEFINITELY: It is a constant used to indicate the 'repeat count' of the trigger is indefinite.
scheduleJob(JobDetail jobDetail, SimpleTrigger simpleTrigger): This method is used to add the JobDetail to the Scheduler, and associate the Trigger with it.
OutPut:
init:
deps-jar:
compile-single:
run-single:
log4j:WARN No appenders could be found for logger (org.quartz.simpl.SimpleThreadPool).
log4j:WARN Please initialize the log4j system properly.
Hello World -- Executed on : Mon Apr 14 22:52:25 IST 2008
Hello World -- Executed on : Mon Apr 14 22:53:25 IST 2008
Hello World -- Executed on : Mon Apr 14 22:54:25 IST 2008
Hello World -- Executed on : Mon Apr 14 22:55:25 IST 2008
Monday, April 7, 2008
Quartz Job Scheduler -- Part 1 (Setting up development project in Netbeans 6.1 beta)
Step 1 :Run Netbeans IDE and create a New Java project opening the new project creation wizard like below.

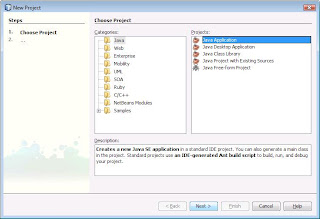
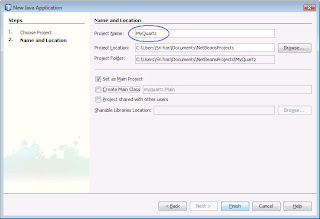
Step 4: The below table explains the files inside the extracted archive.
Files/Directory | Purpose |
quartz-all- | Quartz library includes the core Quartz components and all optional packages. If you are using this library then no other quartz-*.jars need to include. |
quartz- | core Quartz library. |
quartz-jboss- | optional JBoss Quartz extensions such as the Quartz startup MBean, QuartzService. |
quartz-oracle- | optional Oracle specific Quartz extensions such as the OracleDelegate |
quartz-weblogic- | optional WebLogic specific Quartz extensions such as the WebLogicDelegate |
build.xml | an "ANT" build file, for building Quartz. |
docs | root directory of all documentation |
docs/wikidocs | the main documentation for Quartz. Start with the "index.html" |
docs/dbTables | sql scripts for creating Quartz database tables in a variety of different databases. |
src/java/org/quartz | the main package of the Quartz project, containing the 'public' (client-side) API for the scheduler |
src/java/org/quartz/core | a package containing the 'private' (server-side) components of Quartz. |
src/java/org/quartz/simpl | this package contains simple implementations of Quartz support modules (JobStores, ThreadPools, Loggers, etc.) that have no dependencies on external (third-party) products. |
src/java/org/quartz/impl | this package contains implementations of Quartz support modules (JobStores, ThreadPools, Loggers, etc.) that may have dependencies on external (third-party) products - but may be more robust. |
src/java/org/quartz/utils | this package contains some utility/helper components used through-out the main Quartz components. |
src/examples/org/quartz | this directory contains some examples usage of Quartz. |
webapp | this directory contains a simple web-app for managing Quartz schedulers. |
lib | this directory contains all third-party libraries that are needed to use all of the features of Quartz. |
Step 7: Now the Development Environment is ready.
Thursday, March 20, 2008
Collection of Jars in One place
http://www.java2s.com/Code/Jar/CatalogJar.htm
Highlights of NetBeans 6.1
* JavaScript support such as semantic highlighting, code completion, type analysis, quick fixes, semantic checks and refactoring;
* Performance enhancements including faster startup and code completion;
* Spring framework support with features such as configuration file support, code completion and hyperlinks to speed navigation;
* New MySQL support in the Database Explorer to make it easier to create, launch and view MySQL databases;
* Significant enhancements to the Ruby/JRuby support, including a new Ruby platform manager, support for the latest version of Rails and new hints and quick fixes in the editor;
* Beta support for the ClearCase version control system - made available as a plugin from the Update Center.
download from --> http://dlc.sun.com.edgesuite.net/netbeans/6.1/beta/